Mastering Generics in TypeScript - From Basics to Real-World Use
Generics in TypeScript: A deep dive into the concept of generics, their benefits, and how to use them effectively in your TypeScript projects.
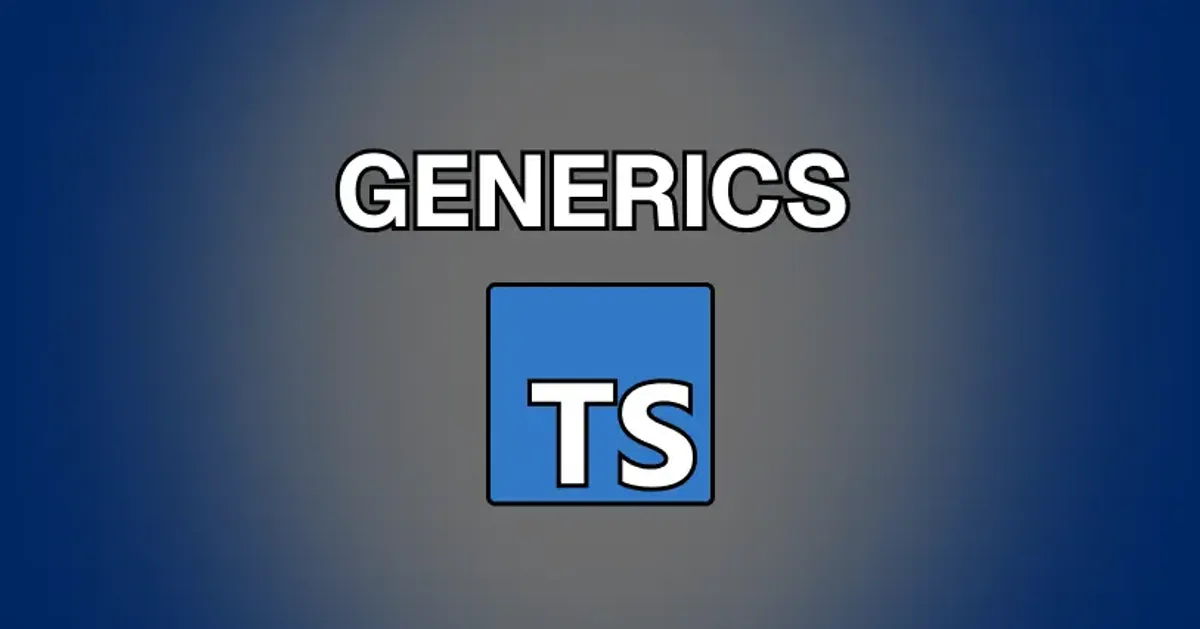
TypeScript is a powerful language, and one of its most versatile features is generics. Whether you’re creating reusable utility functions, managing API responses, or designing robust systems for games or UI components—generics help you write flexible, type-safe code that scales beautifully.
But let’s be honest: at first glance, generics can feel abstract or overly academic. That’s why in this guide, we’ll demystify them through hands-on examples that build up from foundational concepts to real-world web and game development use cases.
These are the topics we will cover in this article:
Basics of Generics
Reusable Functions
Let’s keep it brief: Generics allow us to create functions that work with different types while maintaining type safety. This means you can write a function that can accept a number, string, or any other type without losing the benefits of TypeScript’s type checking.
function identity<T>(value: T): T {
return value
}
const num = identity(42) // Type inferred as number
const str = identity('Hello') // Type inferred as string
We use the T
notation to represent a generic type. This is a placeholder that will be replaced with the actual type when the function is called.
Next you will find the first challenge, which is a simple one. The goal is to create a function that takes a value of any type and returns an array containing that value.
Challenge: Write a function called wrapInArray
that takes a value of any type and returns an array containing that value.
function wrapInArray<T>(value: T): T[] {
return [value]
}
const numArray = wrapInArray(5) // number[]
const strArray = wrapInArray('hello') // string[]
const objArray = wrapInArray({ name: 'John' }) // { name: string }[]
const nestedArray = wrapInArray([1]) // number[][]
const nestedArray2 = wrapInArray([1, 2]) // number[][]
Generic Interfaces
Generics are not just for functions; they can also be used in interfaces. This is particularly useful when you want to define a structure that can work with various types.
interface Box<T> {
value: T
}
const numberBox: Box<number> = { value: 100 }
const stringBox: Box<string> = { value: 'TypeScript' }
In this example, we define a Box
interface that can hold a value of any type. When we create instances of Box
, we specify the type we want to use.
This is a great way to create reusable data structures that can adapt to different types.
Challenge: Create a generic interface called Pair
that holds two values of the same type.
interface Pair<T> {
first: T
second: T
}
const numberPair: Pair<number> = { first: 1, second: 2 }
const stringPair: Pair<string> = { first: 'hello', second: 'world' }
const booleanPair: Pair<boolean> = { first: true, second: false }
Generic Constraints
Sometimes, you want to restrict the types that can be used with generics. This is where constraints come in handy. You can specify that a type must extend a certain interface or class.
function printLength<T extends { length: number }>(item: T): void {
console.log(`Length: ${item.length}`)
}
printLength('Hello') // Length: 5
printLength([1, 2, 3]) // Length: 3
printLength({ length: 10 }) // Length: 10
// printLength(42) // Error: Argument of type '42' is not assignable to parameter of type '{ length: number; }'
In this example, the printLength
function can only accept types that have a length
property. This is useful when you want to ensure that the generic type has certain characteristics.
Challenge: Create a function mergeObjects
that merges two objects but ensures that both arguments are objects.
function mergeObjects<T extends object, U extends object>(a: T, b: U): T & U {
return { ...a, ...b }
}
const obj1 = { name: 'John' }
const obj2 = { age: 30 }
const merged = mergeObjects(obj1, obj2) // { name: string, age: number }
console.log(merged)
Generic Classes
Generics can also be applied to classes, allowing you to create data structures that can hold different types.
class DataStore<T> {
private data: T[] = []
add(item: T): void {
this.data.push(item)
}
getAll(): T[] {
return this.data
}
}
const numberStore = new DataStore<number>()
numberStore.add(1)
numberStore.add(2)
numberStore.add(3)
console.log(numberStore.getAll()) // [1, 2, 3]
const stringStore = new DataStore<string>()
stringStore.add('A')
stringStore.add('B')
stringStore.add('C')
console.log(stringStore.getAll()) // ['A', 'B', 'C']
In this example, we create a DataStore
class that can hold items of any type. The add
method allows us to add items, and the getAll
method retrieves all items in the store.
This is useful for creating collections or repositories that can work with different types of data.
Challenge: Create a KeyValueStore
class that stores key-value pairs, where both key and value are of generic types.
class KeyValueStore<K, V> {
private store: Map<K, V> = new Map()
set(key: K, value: V): void {
this.store.set(key, value)
}
get(key: K): V | undefined {
return this.store.get(key)
}
}
// Expected:
const store = new KeyValueStore<string, number>()
store.set('age', 30)
console.log(store.get('age')) // 30
As you can see, we create a KeyValueStore
class that uses two generic types: K
for the key and V
for the value. The set
method allows us to add key-value pairs, and the get
method retrieves the value associated with a given key.
Generic Type Aliases
You can also create type aliases using generics. When we talk about type aliases, we mean creating a new name for an existing type. This is useful for creating more readable and reusable types.
type Response<T> = {
data: T
status: number
}
type User = {
id: number
name: string
}
type UserResponse = Response<User>
const userResponse: UserResponse = {
data: { id: 1, name: 'John Doe' },
status: 200,
}
In this example, we create a Response
type alias that can hold any type of data. We then create a UserResponse
type that uses the Response
type with a specific User
type.
This is useful for creating reusable types that can adapt to different data structures.
Challenge: Create a type alias called ApiResponse
that represents an API response with a generic data type and a status code.
type ApiResponse<T> = {
data: T
statusCode: number
}
type User = {
id: number
name: string
}
type UserResponse = ApiResponse<User>
const userResponse: UserResponse = {
data: { id: 1, name: 'John Doe' },
statusCode: 200,
}
Generic Utility Types
TypeScript provides several built-in utility types that leverage generics to make your life easier. Some of the most commonly used ones include:
Partial<T>
: Makes all properties ofT
optional.Required<T>
: Makes all properties ofT
required.Readonly<T>
: Makes all properties ofT
read-only.Record<K, T>
: Creates an object type with keys of typeK
and values of typeT
.
interface User {
id: number
name: string
}
const partialUser: Partial<User> = { name: 'Alice' } // Some properties are optional
const readonlyUser: Readonly<User> = { id: 1, name: 'Bob' }
// Record<K, T> creates a type with a set of properties K of type T.
const users: Record<number, User> = {
1: { id: 1, name: 'John' },
2: { id: 2, name: 'Doe' },
}
console.log(partialUser, readonlyUser, users)
In this example, we use Partial
to create a type where all properties of User
are optional. We also use Readonly
to create a type where all properties of User
are read-only. Finally, we use Record
to create an object type with numeric keys and User
values.
Challenge: Define a generic type Nullable that makes all properties of a given type nullable.
type Nullable<T> = { [P in keyof T]: T[P] | null }
interface User2 {
id: number
name: string
}
const nullableUser: Nullable<User2> = { id: null, name: null }
console.log(nullableUser)
Web and Game Development Use Cases
Generics are not just theoretical concepts; they have practical applications in web and game development. Here are some real-world scenarios where generics shine:
API Responses
When working with APIs, you often deal with various data structures. Generics allow you to create reusable types for API responses.
interface ApiResponse<T> {
success: boolean
data: T
error?: string
}
async function fetchData<T>(url: string): Promise<ApiResponse<T>> {
try {
const response = await fetch(url)
const data: T = await response.json()
return { success: true, data }
} catch (error) {
return { success: false, error: (error as Error).message, data: null as T }
}
}
type User = {
id: number
name: string
}
fetchData<User>('https://api.example.com/user/1').then((res) => {
if (res.success) {
console.log(res.data.name)
} else {
console.error(res.error)
}
})
In this example, we create a generic ApiResponse
interface that can hold any type of data. The fetchData
function uses this interface to return a type-safe API response.
This is particularly useful when working with APIs that return different data structures based on the endpoint.
Challenge: Modify fetchData
so that it accepts a transform function to process the response before returning it.
async function fetchData2<T, R>(
url: string,
transform: (data: T) => R,
): Promise<ApiResponse<R>> {
try {
const response = await fetch(url)
const rawData: T = await response.json()
const transformedData = transform(rawData)
return { success: true, data: transformedData }
} catch (error) {
return { success: false, error: (error as Error).message, data: null as R }
}
}
type User = {
id: number
name: string
}
type UserResponse = {
userId: number
userName: string
}
const transformUser = (user: User): UserResponse => ({
userId: user.id,
userName: user.name,
})
fetchData2<User, UserResponse>(
'https://api.example.com/user/1',
transformUser,
).then((res) => {
if (res.success) {
console.log(res.data.userName)
// Output: User's name (e.g., "John Doe")
} else {
console.error(res.error)
}
})
Another use case can be to create a function to abstract the try/catch logic when making API calls. This is very much based in Theo’s approach.
type Success<T> = {
data: T
error: null
}
type Failure<E> = {
data: null
error: E
}
type Result<T, E = Error> = Success<T> | Failure<E>
// Main wrapper function
export async function tryCatch<T, E = Error>(
promise: Promise<T>,
): Promise<Result<T, E>> {
try {
const data = await promise
return { data, error: null }
} catch (error) {
return { data: null, error: error as E }
}
}
// Usage example
async function fetchUser(userId: number): Promise<User> {
const response = await fetch(`https://api.example.com/user/${userId}`)
if (!response.ok) {
throw new Error('Failed to fetch user')
}
return response.json()
}
const result = await tryCatch(fetchUser(1))
if (result.error) {
console.error('Error fetching user:', result.error)
} else {
console.log('Fetched user:', result.data)
}
In this example, we create a tryCatch
function that takes a promise and returns a result object containing either the data or an error. This is useful for handling API calls in a more elegant way.
This approach can be extended to handle different types of errors, such as network errors or validation errors, by using a generic type for the error parameter.
This allows you to create a more flexible and reusable error handling mechanism.
Dynamic Component Props in UI Libraries
Let’s imagine Shadcn does not exist, and we want to create a component library with React that allows us to create dynamic components with different props. Generics can help us achieve this. Let’s start with a button component.
interface ButtonProps<T extends React.ElementType> {
as?: T;
children: React.ReactNode;
props?: React.ComponentProps<T>;
}
const Button = <T extends React.ElementType = "button">({
as,
children,
...props
}: ButtonProps<T>) => {
const Component = as || "button";
return <Component {...props}>{children}</Component>;
};
// Usage
<Button as="a" href="https://example.com">Click Me</Button>;
<Button as="button" onClick={() => alert("Clicked!")}>Click Me</Button>;
<Button as="div" className="custom-class">Click Me</Button>; // this will work too in this version, actually
Challenge: Modify the Button component so that it inherits all valid props from the specified as component.
type ButtonProps<T extends React.ElementType> = {
as?: T;
} & React.ComponentPropsWithoutRef<T>;
const Button = <T extends React.ElementType = "button">({
as,
children,
...props
}: ButtonProps<T>) => {
const Component = as || "button";
return <Component {...props}>{children}</Component>;
};
<Button as="a" href="https://example.com">Click Me</Button>;
<Button as="button" onClick={() => alert("Clicked!")}>Click Me</Button>;
Thanks to this approach, we do not need to pass the props
to the component, as they are automatically inferred from the as
prop. This is a powerful way to create reusable components that can adapt to different use cases.
Generic Entity Manager for Game Objects (Game Development)
class Entity<T> {
constructor(public data: T) {}
update(changes: Partial<T>): void {
this.data = { ...this.data, ...changes }
}
}
// Example usage
interface Player {
name: string
health: number
}
const player = new Entity<Player>({ name: 'Hero', health: 100 })
player.update({ health: 80 })
console.log(player.data) // { name: "Hero", health: 80 }
In this example, we create a generic Entity
class that can hold any type of data. The update
method allows us to update the entity’s data while maintaining type safety. This is useful for creating game objects that can have different properties and behaviors.
Challenge: Modify Entity
to include an id property and add a reset method that restores the original state.
class Entity<T> {
private originalData: T;
public id: string;
constructor(public data: T, id?: string) {
this.originalData = { ...data };
this.id = id || crypto.randomUUID();
}
update(changes: Partial<T>): void {
this.data = { ...this.data, ...changes };
}
reset(): void {
this.data = { ...this.originalData };
}
}
Example usage
interface Player {
name: string;
health: number;
}
const player = new Entity<Player>({ name: "Hero", health: 100 });
player.update({ health: 80 });
console.log(player.data); // { name: "Hero", health: 80 }
player.reset();
console.log(player.data); // { name: "Hero", health: 100 }
Event Bus Pattern
In game development, you often need to communicate between different parts of your application. The Event Bus pattern is a great way to achieve this, and generics can help you create a type-safe event system.
type EventCallback<T> = (data: T) => void
class EventBus {
private events: Map<string, EventCallback<any>[]> = new Map()
on<T>(event: string, callback: EventCallback<T>): void {
if (!this.events.has(event)) {
this.events.set(event, [])
}
this.events.get(event)!.push(callback)
}
emit<T>(event: string, data: T): void {
this.events.get(event)?.forEach((callback) => callback(data))
}
}
// Example usage
const bus = new EventBus()
bus.on<string>('message', (data) => {
console.log(`Received message: ${data}`)
})
bus.on<number>('number', (data) => {
console.log(`Received number: ${data}`)
})
bus.emit('message', 'Hello, World!')
bus.emit('number', 42)
Challenge: Modify the EventBus
so that each event can have multiple listeners, and implement a removeListener method
type EventCallback<T> = (data: T) => void
class EventBus {
private events: Map<string, EventCallback<any>[]> = new Map()
on<T>(event: string, callback: EventCallback<T>): void {
if (!this.events.has(event)) {
this.events.set(event, [])
}
this.events.get(event)!.push(callback)
}
emit<T>(event: string, data: T): void {
this.events.get(event)?.forEach((callback) => callback(data))
}
removeListener<T>(event: string, callback: EventCallback<T>): void {
if (this.events.has(event)) {
this.events.set(
event,
this.events.get(event)!.filter((cb) => cb !== callback),
)
}
}
}
// Example usage
const bus = new EventBus()
const onScoreUpdate = (score: number) => console.log(`Score: ${score}`)
bus.on('scoreUpdated', onScoreUpdate)
bus.emit('scoreUpdated', 100) // "Score: 100"
bus.removeListener('scoreUpdated', onScoreUpdate)
bus.emit('scoreUpdated', 150) // No output since listener is removed
Inventory System
In game development, you often need to manage inventories. Generics can help you create a flexible inventory system that can hold different types of items.
interface Item {
id: string
name: string
}
class Inventory<T extends Item> {
private items: T[] = []
add(item: T): void {
this.items.push(item)
}
remove(id: string): void {
this.items = this.items.filter((item) => item.id !== id)
}
list(): T[] {
return this.items
}
}
interface Weapon extends Item {
damage: number
}
const sword: Weapon = { id: 'sword_01', name: 'Iron Sword', damage: 15 }
const inventory = new Inventory<Weapon>()
inventory.add(sword)
console.log(inventory.list()) // [{ id: 'sword_01', name: 'Iron Sword', damage: 15 }]
inventory.remove('sword_01')
console.log(inventory.list()) // []
Challenge: Modify the Inventory
class to support stacking identical items with a quantity property.
interface Item {
id: string
name: string
}
class Inventory<T extends Item> {
private items: Map<string, { item: T; quantity: number }> = new Map()
add(item: T, quantity: number = 1): void {
if (this.items.has(item.id)) {
this.items.get(item.id)!.quantity += quantity
} else {
this.items.set(item.id, { item, quantity })
}
}
remove(id: string, quantity: number = 1): void {
if (this.items.has(id)) {
const entry = this.items.get(id)!
entry.quantity -= quantity
if (entry.quantity <= 0) {
this.items.delete(id)
}
}
}
list(): { item: T; quantity: number }[] {
return Array.from(this.items.values())
}
}
// Example usage
interface Weapon extends Item {
damage: number
}
const sword: Weapon = { id: 'sword_01', name: 'Iron Sword', damage: 15 }
const inventory = new Inventory<Weapon>()
inventory.add(sword, 2)
console.log(inventory.list()) // [{ item: { id: "sword_01", name: "Iron Sword", damage: 15 }, quantity: 2 }]
inventory.remove('sword_01', 1)
console.log(inventory.list()) // [{ item: { id: "sword_01", name: "Iron Sword", damage: 15 }, quantity: 1 }]
inventory.remove('sword_01', 1)
console.log(inventory.list()) // []
In this example, we create an Inventory
class that can hold items of any type. The add
method allows us to add items, and the remove
method removes items by their ID. The list
method retrieves all items in the inventory.
Conclusion
Generics are a powerful feature of TypeScript that can help you write flexible, reusable, and type-safe code. By understanding the basics of generics and how to apply them in real-world scenarios, you can take your TypeScript skills to the next level. Whether you’re working on web applications, game development, or any other type of software, generics can help you create more robust and maintainable code. If you have any questions or want to share your own experiences with generics, feel free to leave a comment below. Happy coding!
Questions about Generics in TypeScript
Share article