Svelte vs React: A Technical Comparison
Learn the key differences between React and Svelte 5 with a focus on state management, prop drilling, side effects, computed values, and more. Understand how these frameworks handle essential features and which one suits your development needs in 2024
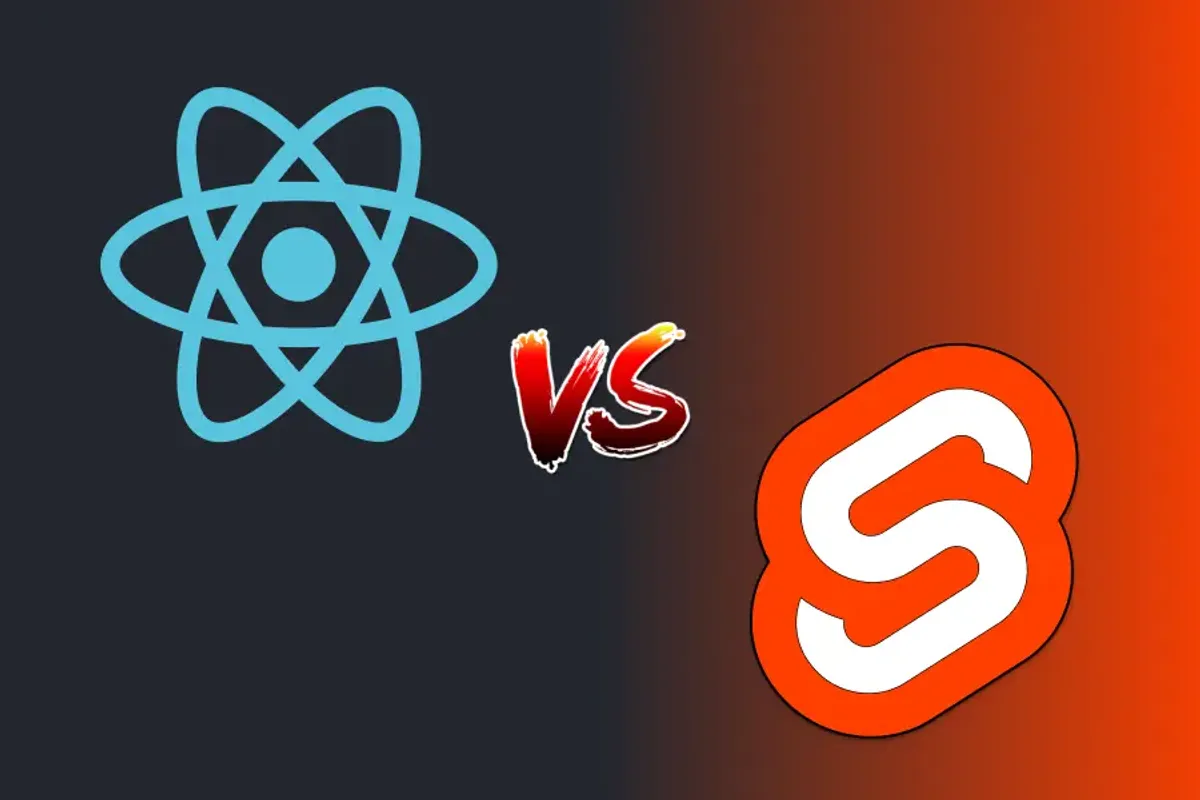
React and Svelte are two of the most popular JavaScript libraries/frameworks, each boasting unique features and a dedicated community. They have been my favourite tools for the last years and as the landscape of front-end development continues to evolve, it’s important to understand how these frameworks compare. This article will explore the differences between Svelte and React, including performance, learning curve, community, and use cases.
These are the topics we will cover in this article:
What is Svelte?
Svelte is an innovative front-end framework that takes a unique approach to building user interfaces. Unlike traditional frameworks like React or Vue, Svelte shifts the bulk of the work to the compile stage, meaning that there’s no virtual DOM. The result is faster performance and more optimized code.
Svelte’s focus on reactivity, minimal boilerplate, and its “write less, do more” philosophy have made it a favorite among developers looking for a modern, efficient way to build web applications. The latest version is Svelte 5, which introduces new features like Runes and Snippets, making it even more powerful and versatile. Check out my article on the new features in Svelte 5 to learn more.
What is React?
React, developed by Facebook in 2013, is a powerful and widely adopted JavaScript library for building complex user interfaces. React popularized the use of the virtual DOM and component-based architecture, making it one of the most trusted solutions for scalable web apps.
The React ecosystem is vast, and its community is constantly growing. React’s focus on reusability, components, and its compatibility with other libraries make it a versatile tool for almost any project.
What we are going to do know is to compare both libraries in different aspects.
You will notice that sometimes I am using the term library
and sometimes
framework
. This is because React is a library and Svelte is a framework. The
way I see it, the main difference is that a framework provides a structure for
your code, while a library is a collection of functions that you can call to
perform tasks. Next.js is a framework built on top of React, for example. And
SvelteKit is a framework built on top of the Svelte framework.
React hooks vs Svelte reactive state
React uses the useState
hook for managing local component state. This hook allows you to create state variables and update them using setter functions. It provides a straightforward way to manage the internal state of a component but can become cumbersome in more complex applications.
// react 18
import { useState } from 'react'
function Counter() {
const [count, setCount] = useState(0)
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
)
}
Svelte 4 and Svelte 5, on the other hand, offer a reactive $ syntax for managing state without needing to explicitly create hooks or setters. Let’s see how the same counter example looks in Svelte:
// svelte 4
<script>
let count = 0
</script>
<div>
<p>Count: {$count}</p>
<button on:click={() => count += 1}>Increment</button>
</div>
// svelte 5
<script>
let count = $state(0)
</script>
<div>
<p>Count: {count}</p>
<button onclick={() => count += 1}>Increment</button>
</div>
This reactivity model makes Svelte’s state management simpler and more intuitive, as you don’t need to rely on hooks or external state management libraries for basic state handling.
Understanding prop drilling in React and Svelte 5
React and Svelte often require prop drilling, which involves passing props through multiple layers of components to reach the desired child. This can make the codebase difficult to maintain as your component hierarchy grows, but sometimes you will have to spot the point where you need to refactor your code to avoid prop drilling and use another strategy.
function ParentComponent() {
const user = { name: 'John Doe' }
return <ChildComponent user={user} />
}
function ChildComponent({ user }) {
return <GrandChildComponent user={user} />
}
function GrandChildComponent({ user }) {
return <p>{user.name}</p>
}
In Svelte you do not need that much repetition when the name of the prop is the same as the name of the variable you are passing.
// Parent.svelte
<script>
let user = $state({ name: 'John Doe' })
</script>
<ChildComponent {user} />
// ChildComponent.svelte
<script>
let { user }= $props()
</script>
<GrandChildComponent {user} />
// GrandChildComponent.svelte
<script>
let { user }= $props()
</script>
<div>{user.name}</div>
It is also worth mentioning that alternative strategies like Context API or global stores can be used to avoid prop drilling in both React and Svelte. Check more about this in the following links:
Side Effects
In React, side effects are handled using the useEffect
hook. The useEffect
hook is powerful but requires careful management of dependencies to avoid unnecessary re-renders or infinite loops.
import { useEffect } from 'react'
function App() {
useEffect(() => {
console.log('Component mounted or updated')
return () => console.log('Cleanup on unmount')
}, [])
return <div>Hello, World!</div>
}
Svelte 5 provides a similar way to handle side effects by using the $effect rune.
<script>
let name = 'World';
$effect(() => {
console.log(`Hello, ${name}!`);
return () => console.log('Cleanup on unmount');
});
</script>
The $effect rune allows you to define side effects directly in your component without the need for hooks or external libraries. This makes it easier to manage side effects and keep your codebase clean and concise.
Derived/Computed Values
React does not have a built-in way to handle derived values, so developers usually need to compute values manually within components or use useMemo for optimization purposes.
import { useMemo, useState } from 'react'
function App() {
const [count, setCount] = useState(0)
const doubleCount = useMemo(() => count * 2, [count])
return (
<div>
<p>Double Count: {doubleCount}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
)
}
In Svelte 4, derived values are handled automatically using the $: syntax. This syntax was a bit buggy in Svelte 4 (sometimes the values were not updated when expected), but in Svelte 5, it was replaced by the $derived
rune, which provides a more reliable way to compute values based on other values.
<script>
let count = $state(1);
let doubleCount = $derived(count * 2);
</script>
<div>
<p>Double Count: {doubleCount}</p>
<button onclick={() => count++}>Increment</button>
</div>
The $derived rune makes it easy to compute values based on other values without the need for additional hooks or memoization.
Ecosystem and Community
React has a vast and mature ecosystem with thousands of third-party libraries, components, and tools. It is the most popular front-end framework and benefits from extensive community support and resources. Tools like Redux, React Router, and Next.js extend its capabilities for enterprise-level applications.
Svelte 5, while still growing, has an increasingly vibrant community. It doesn’t have as vast an ecosystem as React but offers SvelteKit as a robust solution for building full-stack web applications, and its simple approach often reduces the need for many external libraries.
Learning Curve
The learning curve for React can be steeper, especially for beginners. Learning JSX, managing state, hooks, and lifecycle methods all add to the complexity. However, once learned, React’s robust ecosystem and versatility make it a powerful tool.
Svelte 5 is considered easier for beginners to pick up. It uses plain HTML, CSS, and JavaScript syntax, and its reactivity model is built directly into the language, which reduces the need for learning complex concepts.
Summary Table
Here is a summary table comparing React and Svelte. I have decided to focus on the latest versions: React 18/19 and Svelte 5.
Feature | React 18/19 | Svelte 5 |
---|---|---|
State Management | Uses useState or a centralized store like Redux or Context API | Built-in reactivity system with runes like $state , but also stores, Context API. |
Prop Drilling | Requires passing props down the component tree like foo={foo} | Automatically passes props to child components like {foo} |
Side Effects | Uses useEffect with dependency array | Uses reactive statements and lifecycle functions for side effects |
Computed Values | Uses memoization (useMemo , useCallback ) or re-renders components to compute values | Uses reactive statements to compute values with $derived |
Learning Curve | Steeper learning curve due to complex concepts like JSX, props, and state management | Easier learning curve with a focus on simplicity and reactivity |
Community | Large and active community with a wide range of libraries and tools | Growing community with a focus on simplicity and performance |
Use Cases | Ideal for complex applications with a need for scalability and reusability | Ideal for small to medium-sized applications with a focus on performance |
Conclusion
React and Svelte are both powerful tools for building modern web applications, each with its own strengths and weaknesses. React is a mature and versatile library with a vast ecosystem, making it ideal for complex applications that require scalability and reusability. Svelte, on the other hand, is a lightweight and efficient framework that simplifies the development process with its reactivity model and minimal boilerplate.
When choosing between React and Svelte, consider the complexity of your project, your familiarity with the library, and your team’s expertise. Both React and Svelte have their place in the front-end development landscape, and the best choice depends on your specific needs and goals, or what you feel like using!
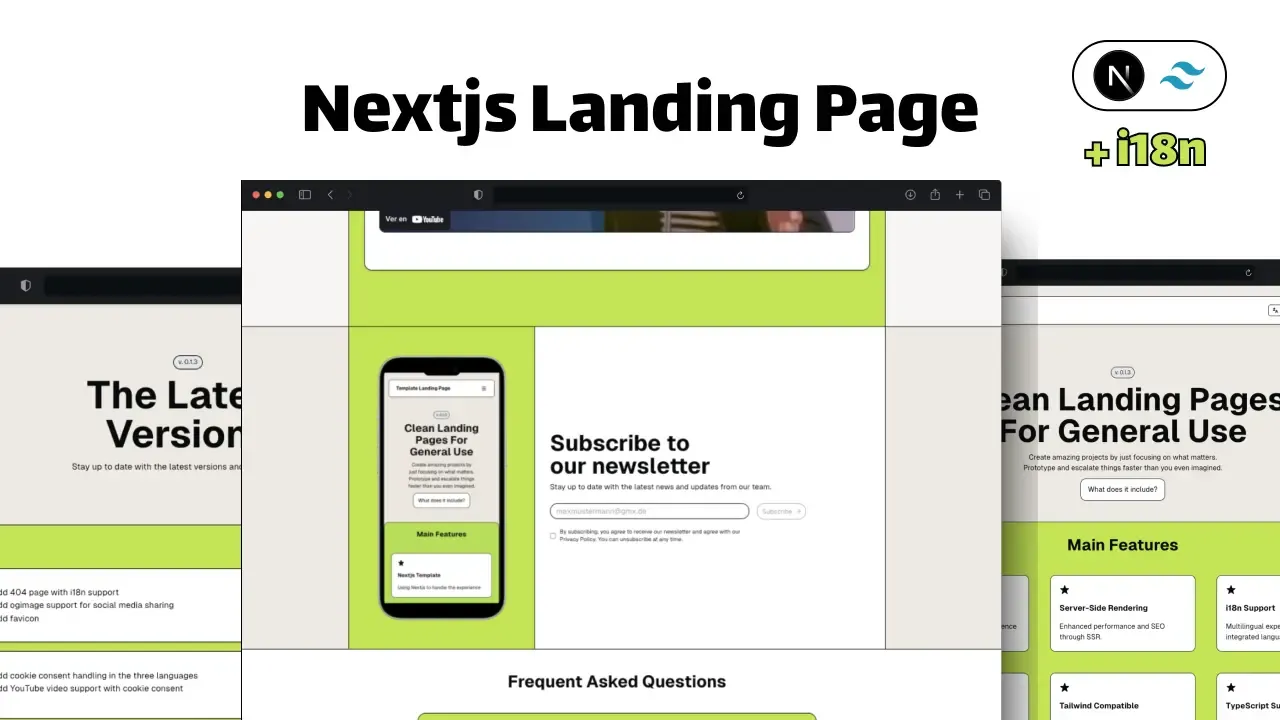
Check out my latest Nextjs 15 template! Done with motion, shadcn, support for several languages, newsletter, etc!
See moreFAQ about Comparison Between React and Svelte
Share article