20+ Common Examples of Technical Debt (and How to Fix Them)
Technical debt slows down development and increases maintenance costs. Learn about 20+ real-world examples of technical debt, their impact, and how to prevent or fix them in your codebase.
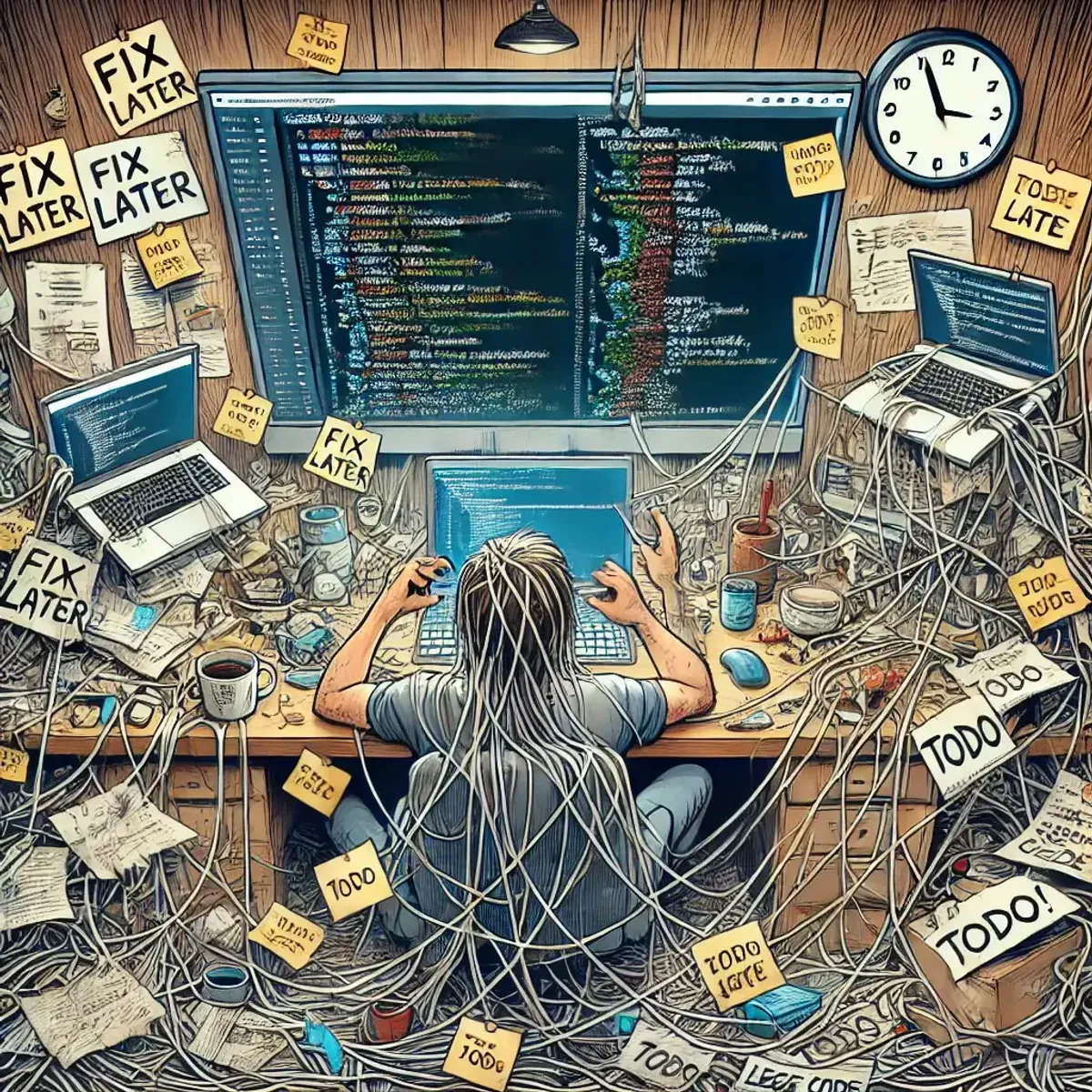
Every developer has faced a moment when they needed to push a feature quickly, knowing that the code could have been cleaner. This trade-off between speed and quality is what we call technical debt—the compromises made in development that lead to long-term inefficiencies.
Like financial debt, technical debt accrues “interest.” The longer you leave it unresolved, the more it impacts code maintainability, scalability, and performance. But not all technical debt is bad! Sometimes, taking shortcuts is necessary for rapid iteration—as long as you have a plan to address them later.
In this article, we’ll explore 20+ real-world examples of technical debt, their impact on development teams, and how you can effectively refactor your code to minimize risks.
Examples of Technical Debt in Software Development
For every single example, we’ll discuss the impact of the technical debt and provide solutions to fix them. Let’s dive in!
1. Hardcoded Values Instead of Configuration Files
- Example: API URLs, database credentials, or feature flags are hardcoded into the source code.
- Debt Impact: Difficult to modify settings without redeploying the code; risk of exposing sensitive data.
- Solution: Use environment variables or configuration management tools like
.env
files.
2. Lack of Error Handling & Logging
- Example: An application catches all errors but doesn’t log them or handle them properly.
- Debt Impact: Debugging production issues becomes difficult; hidden failures cause unexpected behavior.
- Solution: Implement centralized logging with tools like Sentry or Winston and enforce structured error handling.
3. Ignoring Web Accessibility (a11y) Standards
- Example: A website relies on
div
elements for buttons without proper aria attributes. - Debt Impact: Users with disabilities struggle to navigate the site; potential legal consequences.
- Solution: Use semantic HTML and follow WCAG guidelines to improve accessibility from the start.
4. Monolithic Codebase Without Modularization
- Example: A single JavaScript file grows to thousands of lines without clear separation of concerns.
- Debt Impact: Code is difficult to maintain, test, and scale.
- Solution: Refactor into smaller modules or components using modern architectures like microservices or component-driven development.
5. Over-Reliance on Deprecated Libraries or APIs
- Example: A React project still uses
componentWillMount
instead ofuseEffect
or updated lifecycle methods. - Debt Impact: The code may break in future updates, forcing major refactors.
- Solution: Regularly audit dependencies and upgrade libraries using tools like
npm-check
orDependabot
.
6. Insufficient Test Coverage (or No Tests at All!)
- Example: A project lacks unit, integration, or end-to-end tests.
- Debt Impact: Refactoring becomes risky, and regressions occur frequently.
- Solution: Implement a testing strategy using
Vitest/Jest
(for unit tests),Cypress
orPlaywright
(for E2E testing).
7. Tightly Coupled Components in Frontend Applications
- Example: A React component directly fetches data from an API, making it hard to reuse.
- Debt Impact: Changes in data fetching require modifying multiple components.
- Solution: Use separation of concerns with hooks, context, or state management libraries like Redux or Zustand.
8. Inefficient Database Queries (N+1 Query Problem)
- Example: A backend API makes multiple small queries instead of using a JOIN or optimized query.
- Debt Impact: Performance degrades significantly as data grows.
- Solution: Use database profiling tools, indexes, and ORM optimizations to avoid redundant queries.
9. Poor Documentation & Knowledge Silos
- Example: Only one team member understands a complex function, and there’s no documentation.
- Debt Impact: If they leave, the team struggles to maintain or improve the feature.
- Solution: Adopt documentation-first principles using tools like
JSDoc
,Markdown
, orinternal wikis
.
10. Misuse of CSS (Spaghetti Styles or !important Overload)
- Example: Stylesheets are filled with conflicting !important rules, leading to specificity wars.
- Debt Impact: Minor UI changes require hunting through styles, making maintenance painful.
- Solution: Use CSS methodologies like BEM, Tailwind utility classes, or CSS-in-JS solutions.
11. Poorly Designed APIs (Unintuitive Endpoints or Overfetching)
- Example: An API endpoint returns way too much unnecessary data, increasing network load.
- Debt Impact: Clients must filter out unused fields, making API requests slower.
- Solution: Implement
GraphQL
or optimize REST API responses to return only required data.
12. Too Many Global Variables in JavaScript
- Example: A JavaScript project relies on global variables instead of proper module imports.
- Debt Impact: Hard to track where values are changed, increasing the likelihood of bugs.
- Solution: Use ES6 modules (import/export) and avoid polluting the global scope.
13. Overuse of Copy-Paste Code (Code Duplication)
- Example: The same logic appears in multiple places instead of being reused.
- Debt Impact: Fixing a bug in one place doesn’t automatically fix it elsewhere.
- Solution: Refactor repeated logic into reusable functions, hooks, or components. Follow the DRY principle (Don’t Repeat Yourself).
14. Lack of CI/CD Integration (Manual Deployments)
- Example: A team manually deploys changes via FTP or SSH instead of using a CI/CD pipeline.
- Debt Impact: Inconsistent deployments, longer development cycles, and risk of human error.
- Solution: Implement automated CI/CD pipelines using GitHub Actions, GitLab CI, or CircleCI.
15. Unoptimized CSS Selectors (Performance Bottlenecks)
- Example: Using overly complex CSS selectors like div > section > ul > li:first-child.
- Debt Impact: Browser rendering slows down due to inefficient DOM lookups.
- Solution: Use class-based styling (.btn-primary) and avoid deep nested selectors.
16. Overengineering (Overcomplicated Solutions for Simple Problems)
- Example: Implementing a microservices architecture for a simple CRUD application.
- Debt Impact: Increases complexity, making debugging and maintenance harder.
- Solution: Follow YAGNI (You Ain’t Gonna Need It) and avoid unnecessary abstractions.
17. Unused or Bloated Dependencies in a Project
- Example: A React project still has unused dependencies from older versions (
package.json
cluttered). - Debt Impact: Increases bundle size, slows down builds, and introduces security risks.
- Solution: Regularly run
npm prune
,yarn deduplicate
, andnpm audit
to clean dependencies.
18. Lack of Scalability in Database Design
- Example: Using sequential IDs instead of UUIDs in a database schema.
- Debt Impact: Can cause conflicts in distributed systems and makes horizontal scaling harder.
- Solution: Choose database-friendly scalable strategies like UUIDs or sharded keys.
19. Legacy Code That No One Wants to Touch
- Example: A jQuery-heavy project inside a modern React or Vue ecosystem.
- Debt Impact: New developers struggle to integrate modern tools without breaking legacy features.
- Solution: Gradually migrate legacy code, replacing jQuery with native JavaScript or modern frameworks.
20. Overcomplicated State Management in Frontend Apps
- Example: Using Redux for every small state update when
useState
orContext API
would suffice. - Debt Impact: Adds unnecessary boilerplate and complexity to frontend applications.
- Solution: Use lightweight state management solutions like
Zustand
,Jotai
, or evenuseReducer
.
Conclusion: Managing Technical Debt Wisely
Technical debt is inevitable in software development—but how you manage it makes all the difference. Some forms of technical debt are acceptable if they allow a team to ship products faster, but they should be tracked and addressed before they cause bigger problems.
By recognizing these common examples of technical debt, developers and teams can take proactive steps to refactor code, improve maintainability, and build scalable applications without sacrificing long-term quality.
FAQ about Technical Debt
Technical debt refers to the trade-offs developers make to speed up delivery, often at the cost of maintainability, performance, or scalability. Like financial debt, it accumulates "interest" over time if not addressed, making future development more difficult and costly.
Share article