Api-First vs Code-First: Which One Should You Choose?
Learn the key differences between API-First and Code-First approaches in development. Discover their advantages, disadvantages, and how they impact your project design!
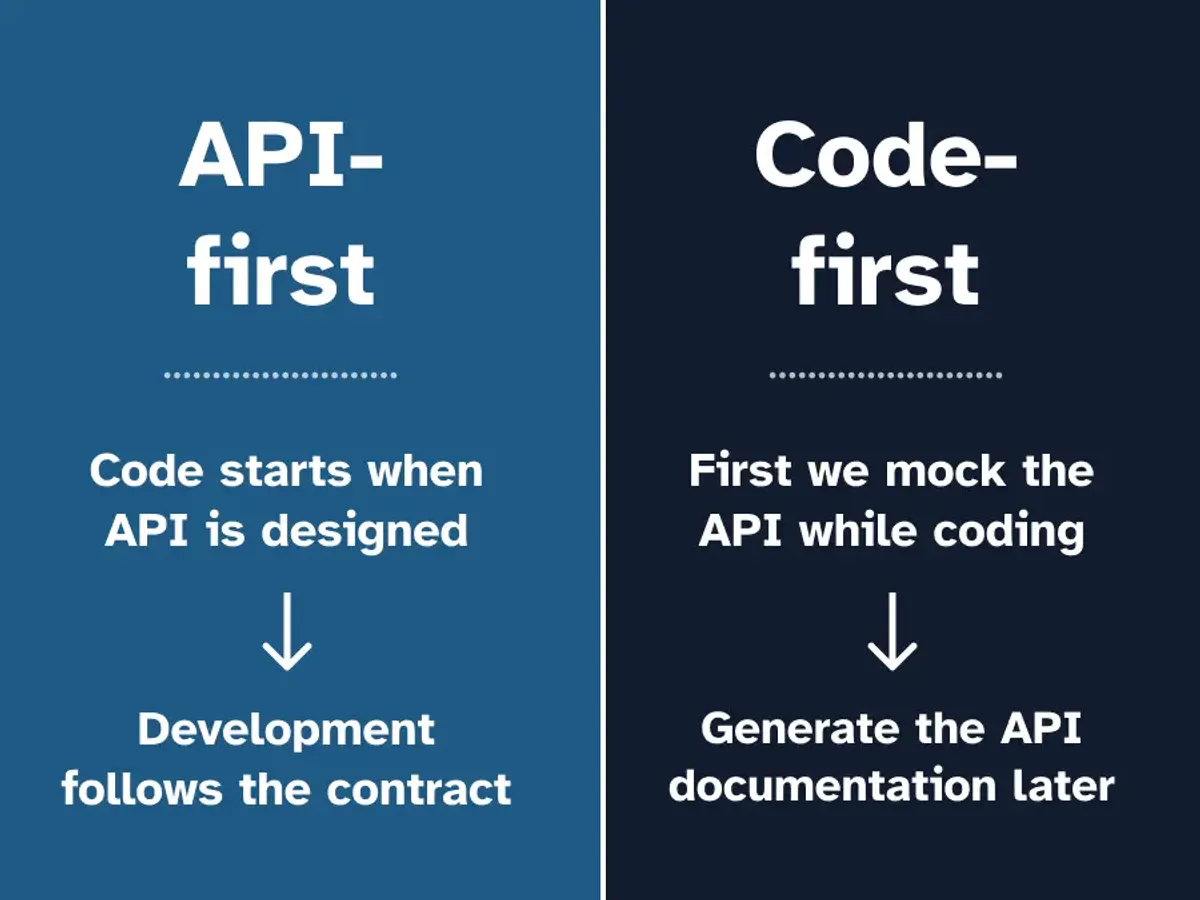
When building modern applications, especially with backend services like APIs, there are two main approaches: API-First
(also known as Design-First) and Code-First
. Both have their unique advantages and disadvantages depending on your project and team setup. This article breaks down these concepts in simple terms, using examples in TypeScript and Node.js to make the comparison clear.
What is the API-First Approach?
In an API-First approach, you start by designing the API. This means that before any code is written, you plan and define the API endpoints, methods, request/response formats, and more. Typically, a specification language like OpenAPI (formerly Swagger) is used to outline the API’s structure. Once the API design is complete, developers use the specification to implement the API or generate code automatically.
Advantages of API-First:
Consistency Across Teams: Since the API is designed upfront, all teams (frontend, backend, etc.) agree on how the API will behave. This avoids miscommunication.
API Documentation from the Start: Tools like Swagger automatically generate API documentation from your API design, which makes it easy to share and understand.
Parallel Development: With the API design ready, frontend and backend teams can work in parallel, saving time.
Scalability: API-First promotes reusability and consistency, making it easier to scale and manage larger systems. Disadvantages of API-First.
Disadvantages of API-First:
Upfront planning: Requires more effort at the beginning to ensure the API design is comprehensive. This can slow down the initial phase.
Rigid: If changes are needed later, you might face some resistance, as the API contract is already established.
Example in TypeScript (API-First)
Here’s how you might define an API contract using OpenAPI in a TypeScript project:
# OpenAPI specification example
openapi: 3.0.0
info:
title: Sample API
version: 1.0.0
paths:
/users:
get:
summary: Get all users
responses:
'200':
description: A list of users
Once this is defined, tools like Swagger Codegen can generate TypeScript server or client code, and the development team follows the design.
What is the Code-First Approach?
In a Code-First approach, developers first write the code, such as backend logic in Node.js or Python, without having a detailed API contract upfront. Once the code is functional, tools can be used to generate the API documentation.
For example, developers might create routes in Node.js, and then document the API afterwards.
Advantages of Code-First:
Quick Start: You can dive straight into coding without needing to define everything upfront. This is great for small projects or MVPs.
Flexible: You can adapt the API as your project evolves, without being locked into a strict contract early on.
Less Planning: Ideal when requirements are not fully clear at the start and you expect the API to evolve frequently.
Disadvantages of Code-First:
Lack of Consistency: Without a clear design upfront, different developers might implement APIs in inconsistent ways.
Documentation is an Afterthought: API documentation often comes after the code, which can lead to incomplete or outdated documentation.
Harder Collaboration: Frontend and backend teams may not have a clear agreement on the API until it’s built, which can lead to delays or rework.
Example in TypeScript (Code-First)
Here’s a basic example of creating an API using Node.js with Express, following the Code-First approach:
import express from 'express'
const app = express()
// Define a route without upfront API design
app.get('/users', (req, res) => {
res.json([
{ id: 1, name: 'John Doe' },
{ id: 2, name: 'Jane Doe' },
])
})
app.listen(3000, () => {
console.log('Server running on port 3000')
})
Once the code is written, you could use tools like Swagger UI to generate the API documentation based on this implementation.
When Should You Use API-First and when Code-First?
API-First
Larger teams: If your team is split between frontend and backend developers, and both need to work simultaneously, API-First ensures everyone is on the same page.
Public APIs: If you’re building an API that others will consume, a well-documented and consistent API is critical.
Long-term projects: API-First provides structure that’s especially beneficial for long-term, complex projects.
Code-First
Small projects or prototypes: If you’re building a small project or prototype that’s likely to evolve quickly, Code-First offers more flexibility.
Solo development: When one person is handling both frontend and backend, the need for upfront API design is less critical, and you can code as you go.
Rapid iterations: If the API needs to change frequently during development, Code-First allows for more adaptability.
FAQ about API-First and Code first approaches
The main difference is in the process. In API-First, the API is designed upfront, and development follows the contract. In Code-First, you write the code first and generate the API documentation afterwards.
Share article